As I’ve mentioned in previous posts, I’ve been taking an awesome course on Node for the last several months. This course has really helped me learn JavaScript concepts that I knew about but didn’t understand.
Last month I explained promises. The basic idea of promises is that node functions happen asynchronously, so promises are like place-savers that wait for either a resolve or reject response.
Here’s an example of a function with a promise:
const add = (a, b) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(a+b)
}, 2000)
})
}
In this function, we’re simply adding two numbers. The setTimeout for two seconds is added to make the function take a little longer, as a real function would.
Here’s what calling the function might look like:
add(1, 2).then((sum) => {
console.log(sum)
})
The add function is called, and then we use the then command to log the result. Using then() means that we’re waiting for the promise to be resolved before we go on.
But what if we needed to call the function a second time? Let’s say we wanted to add two numbers, then we wanted to take that sum and add a third number to it. For this we’d need to do promise chaining. Here’s an example of promise chaining:
add(1, 2).then((sum) => {
add(sum, 3).then((sum2) => {
console.log(sum2)
})
})
The add function is called, and we use the then() command with the sum that is returned. Then we call the function again, and use the then() command once again with the new sum that is returned. Finally, we log the new sum.
This works just fine, but imagine if we had to chain a number of function calls together? It would start to get pretty tricky with all the thens, curly braces and indenting. And this is why async/await was invented!
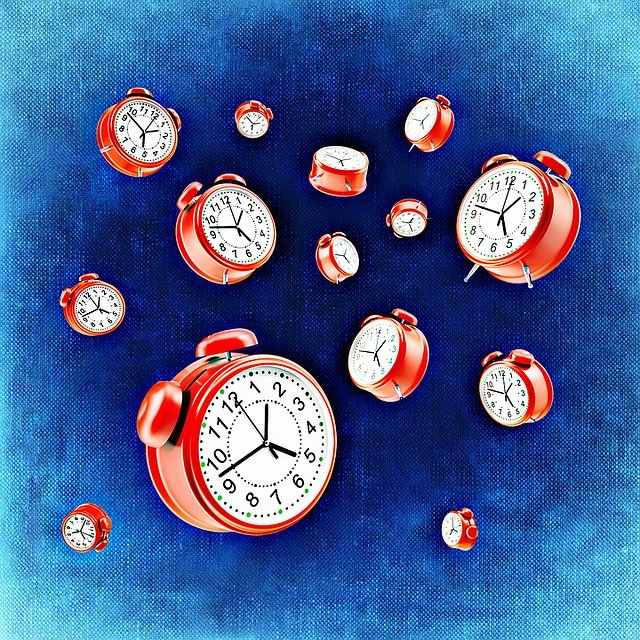
With async/await, you don’t have to chain promises together. You create a new async function call, and then you use the await command when you are calling a function with a promise.
Here’s what the chained promise call would look like if we used async/await instead:
const getSum = async () => {
const sum = await add(1, 2)
const sum2 = await add(sum, 3)
console.log(sum2)
}
getSum()
We’re creating a new async function called getSum, by using this command:
const getSum = async () =>. In that function, we’re first calling the add function with an await, and we’re setting the result of that call to the variable called sum. Then we’re calling the add function again with an await, and we’re setting the result of that call to the variable called sum2. Finally, we’re logging the value of sum2.
Now that the async function has been created, we’re calling it with the getSum() command.
It’s pretty clear that this code is easier to read with async/await! Keep in mind that promises are still being used here; the add() function still returns a promise. But async/await provides a way to call a promise function without having to add in a then() statement.
Now that I understand about async/await, I plan to use it whenever I am doing development or writing test code in Node. I hope you’ll take the time to try out these examples for yourself to see how they work!
Pingback: Five Blogs – 20 July 2020 – 5blogs