One of the things I struggled with when I started learning object-oriented programming (OOP) was the way objects were created and referenced. Things seemed so much simpler to me years ago when a computer program was in one file and you declared all your variables at the top!
But the more I’ve worked with OOP, the more I’ve been able to understand it. And the work I’ve done while taking this awesome Node.js course has helped me even more. I’ve already written a few posts about the great things I’ve learned in Node.js: Async/Await functions, Promises, and Arrow Functions. In this post, I’ll talk about two great ways to write less code: Object Property Shorthand and Object Destructuring.
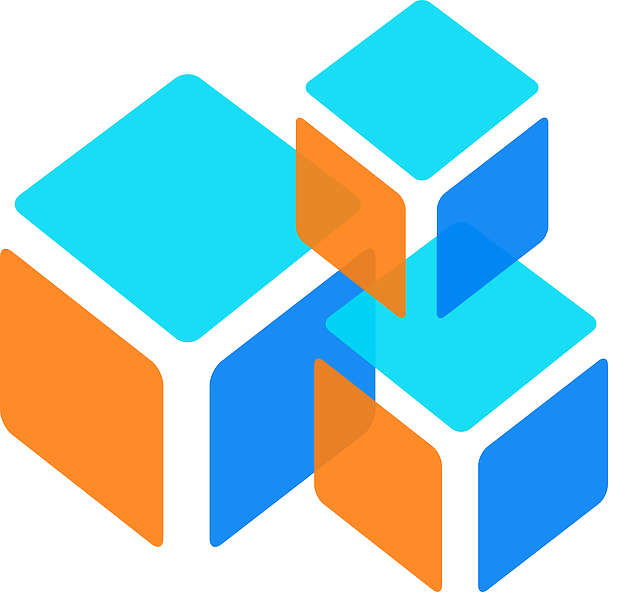
First, let’s take a look at how Object Property Shorthand works. We’ll start with a few variables:
var name = ‘Fluffy’
var type = ‘Cat’
var age = 2
Now we’ll use those variables to create a pet object:
const pet = {
name: name,
type: type,
age: age
}
When we create this object, we are saying that we want the pet’s name to equal the name variable that we set above, the pet’s type to equal the type variable we set above, and the pet’s age to equal the age variable we set above.
But doesn’t it seem kind of silly to have to repeat ourselves in this way? Imagine if the pet object had several more properties: we’d go on adding things like ownerName: ownerName, address: address, and so on. Wouldn’t it be nice to save ourselves some typing?
Good news- we can! If the property name in our object matches the variable name we are setting it to, we can save typing time by doing this:
const pet = {
name,
type,
age
}
That’s a lot easier to type, and to read! If you have Node.js installed, you can try it for yourself by creating a simple file called pet.js. Begin by declaring the variables, then create the object the old way, then add console.log(pet). Run the program with node pet.js, and you should get this response: { name: ‘Fluffy’, type: ‘Cat’, age: 2 }. Now delete the object creation and create the pet the new way. Run the program again, and you should get the same response!
Now let’s take a look at Object Destructuring. We’ll start by creating a new book object:
const book = {
title: ‘Alice in Wonderland’,
author: ‘Lewis Carroll’,
year: ‘1865’
}
The old way of referencing the properties of this book object is to refer to them by starting with “book.” and then adding on the property name. For example:
const title = book.title,
const author = book.author,
const year = book.year
But just as we saw with Object Property Shorthand, this seems a little too repetitive. If the property name is going to match the variable name we want to use, we can set all of the variables like this:
const {title, author, year} = book
What this command is saying is “Take these three properties from book, and assign them to variables that have the same name.”
You can try this out yourself by creating the book object, setting the variables the old way, then adding a log command: console.log(title, author, year). Run the file, and you should see Alice in Wonderland Lewis Carroll 1865 as the response. Then replace the old variable assignments with the new Destructuring way, and run the file again. You should get the same response.
Keep in mind that Shorthand and Destructuring only work if the property name and the variable name match! In our pet example, if we wanted to set a pet property called petAge instead of age, we’d need to do this:
const pet = {
name,
type,
petAge: age
}
And in our book example, if we wanted to use a variable called authorName instead of author, we’d need to do this:
const {title, author:authorName, year} = book
Even if we don’t have a perfect match between all our object properties and variables, it’s still easy to see how much less typing we need to do when we use Shorthand and Destructuring! I hope you’ll find this helpful as you are writing automation code.
Pingback: Five Blogs – 6 September 2020 – 5blogs